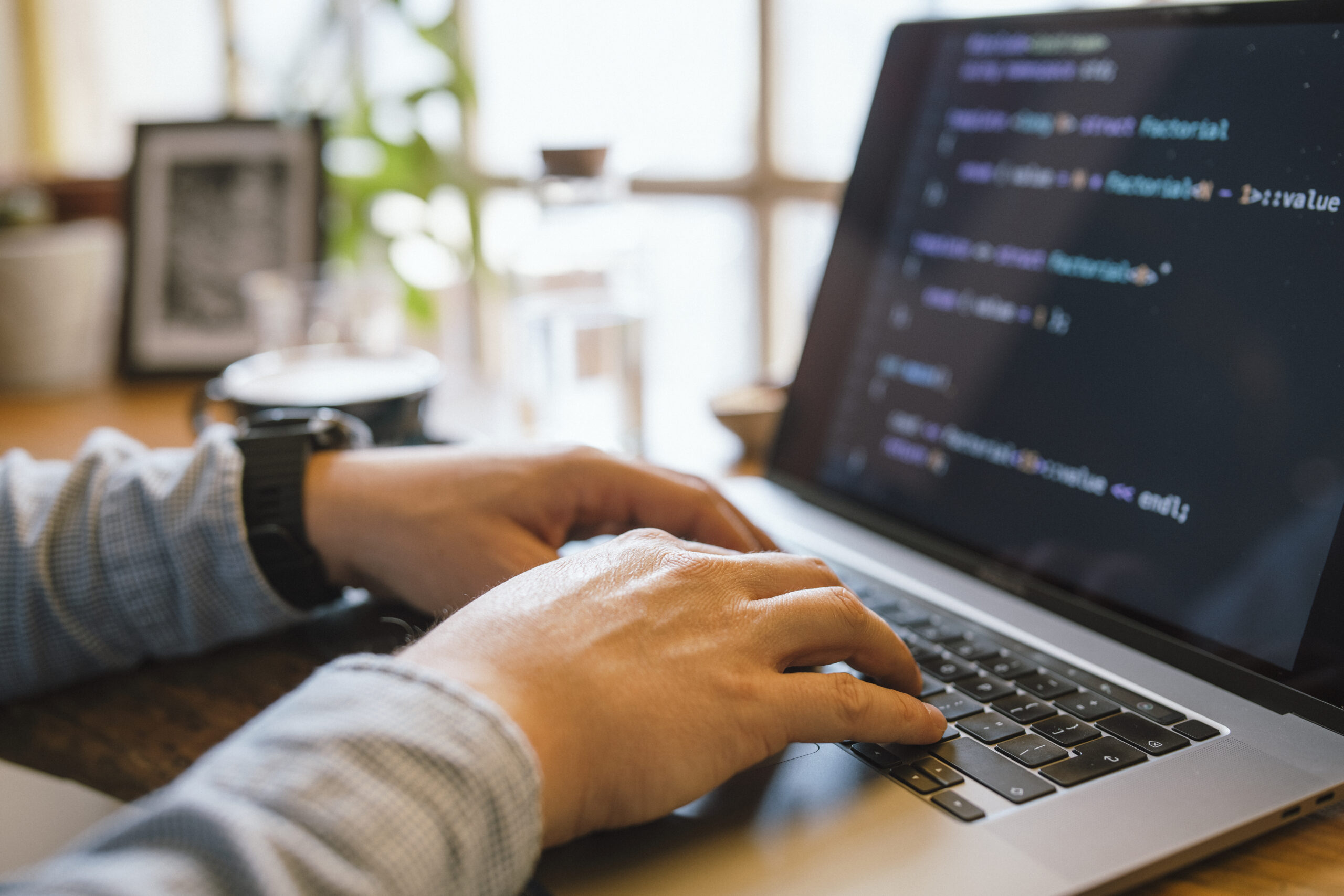
Debugging is one of the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about being familiar with how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can preserve hrs of annoyance and radically improve your productivity. Listed here are a number of methods to aid developers degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest approaches builders can elevate their debugging capabilities is by mastering the equipment they use on a daily basis. Though creating code is a single A part of development, recognizing ways to communicate with it properly in the course of execution is equally important. Modern development environments occur Outfitted with effective debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Acquire, as an example, an Integrated Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of accurately, they let you observe exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They enable you to inspect the DOM, keep track of community requests, view actual-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control more than jogging procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Command methods like Git to comprehend code heritage, obtain the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your development atmosphere in order that when concerns come up, you’re not misplaced at nighttime. The higher you understand your equipment, the more time you are able to invest solving the actual trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping into the code or making guesses, builders need to have to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of probability, generally resulting in wasted time and fragile code modifications.
The initial step in reproducing a challenge is collecting as much context as is possible. Question queries like: What steps led to The difficulty? Which environment was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you've got, the easier it will become to isolate the exact disorders underneath which the bug occurs.
As you’ve collected more than enough details, try to recreate the challenge in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty may be setting-precise — it might take place only on specified working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible circumstance, You should utilize your debugging instruments additional correctly, exam opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance into a concrete problem — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must discover to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by reading through the message thoroughly and in full. Lots of developers, especially when underneath time stress, look at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and recognize them initial.
Crack the error down into areas. Is it a syntax mistake, a runtime exception, or a logic error? Will it level to a selected file and line quantity? What module or functionality induced it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A superb logging approach commences with realizing what to log and at what degree. Typical logging ranges include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during enhancement, Facts for normal functions (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for true difficulties, and FATAL in the event the technique can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your program. Focus on vital functions, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a very well-thought-out logging technique, you can reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technological job—it's a kind of investigation. To correctly identify and repair bugs, developers have to solution the procedure like a detective solving a thriller. This state of mind aids stop working advanced issues into manageable elements and comply with clues logically to uncover the foundation induce.
Get started by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece collectively a clear picture of what’s happening.
Future, variety hypotheses. Check with on your own: What may be triggering this conduct? Have any modifications recently been built into the codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down alternatives and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. Should you suspect a specific functionality or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Fork out near notice to modest particulars. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person error, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Tests
Composing assessments is among the simplest ways to enhance your debugging expertise and Total progress performance. Checks not only assist catch bugs early but additionally serve as a safety net that provides you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you right away know in which to appear, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—troubles that reappear soon after Formerly being preset.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various elements or services interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you need to be aware of its inputs, expected outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to center on fixing the bug and observe your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks will help you reset your head, lower annoyance, and infrequently see The problem from the new viewpoint.
When you are also close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief walk, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious perform within the history.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re stuck, a very good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that point to move all over, stretch, or do a thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it basically contributes to a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking your self several essential issues when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses generally expose blind places with your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring read more concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best builders are not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Every bug you deal with adds a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.